Reporting Crashes in IMVU: Last-Chance Exceptions
So far, our crash reporting is looking pretty comprehensive. But what if there is a crash in the crash reporting itself? Or perhaps a crash on another thread (outside of any
In IMVU's last-chance handler, we do what we can to save the state of the failure to disk. The next time the client starts, if failure data exists, the client submits it to the server. (Assuming the customer tries to run the client again.) Here is is the last-chance handler's implementation:
__try ... __except
blocks)? Or, heaven forbid, we somehow cause Python itself to crash? In all of these situations, we can't count on our Python crash reporting code to handle the error.
There are a couple ways to report these failures, and IMVU chose to implement a last-chance exception handler with SetUnhandledExceptionFilter. This handler runs whenever a structured exception bubbles out of any thread in the process. Unfortunately, you can't run arbitrary code in the handler - maybe your process's heap is corrupted and further attempts to allocate will cause another access violation.
By the way, the last-chance handler is your opportunity to run code just before the Windows "this program has performed an illegal operation" dialog.
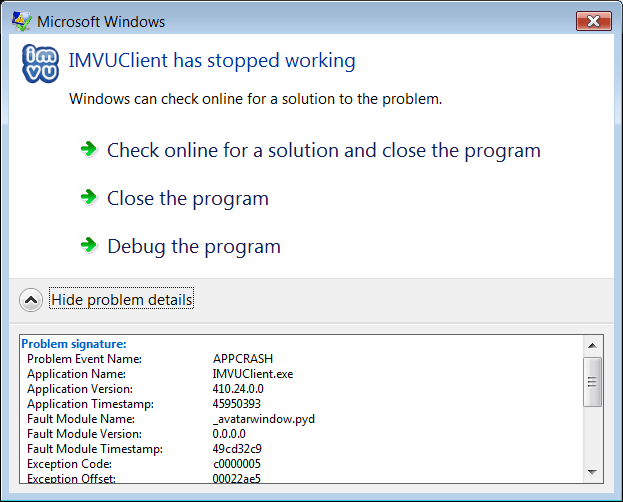
LONG WINAPI handleLastChanceException( EXCEPTION_POINTERS* ExceptionInfo ) { DWORD error = writeMiniDump( ExceptionInfo); if (error) { CRASHLOG("Failed to write minidump"); } // Try to record some additional system // info if we can. // Show the application error dialog. return EXCEPTION_CONTINUE_SEARCH; }Pretty easy! Again, try to restrict yourself to system calls in your last-chance handler. When we first implemented this, we found out that the IMVU client was crashing a ton and we didn't even know! Were I to start over from scratch, I'd implement the broadest crash detection possible first, and then implement narrower, more-detailed detection as necessary. Next time I'll talk about an unexpected shortcoming of this implementation. (Can you guess what it is?)
[...] Reporting Crashes in IMVU: Last-Chance Exceptions [...]
my uploader dont work it want read my averta name or pass word and all my things or gone please help asap