IMVU Crash Reporting: Plugging the VC++ Runtime's Escape Hatches
Modern software is built on top of piles of third-party components. The holy grail of reusable code has manifested in the form of an open source ecosystem. That said, in a startup, you rarely have time to audit the third-party components you use. These third-party components might be ridiculous enough to call abort()
on error. It may sound scary, but with a fixed amount of work, you can turn these calls into reported structured exceptions.
Unfortunately, the Visual C++ Runtime provides several functions that abnormally terminate the process without running our crash handlers. As a bonus, they usually include user-incomprehensible dialog boxes. Let's see:
- abort()
- "pure virtual" method calls
- throwing an exception from a destructor during unwind
- stack buffer overflow (with /GS enabled)
- standard C++ library index/iterator error with checked iterators enabled
Since you can never prove that you've implemented crash reporting for every way a third-party component can bypass your crash reporting, I'm just going to cover the ones we've implemented:
abort()

Turning abort()
into a structured exception is pretty straightforward. A quick read of the CRT source shows that abort()
runs SIGABRT's installed signal handler. It's easy enough to install a custom handler that raises a structured exception:
void __cdecl onSignalAbort(int code) { // It's possible that this signal handler will get called twice // in a single execution of the application. (On multiple threads, // for example.) Since raise() resets the signal handler, put it back. signal(SIGABRT, onSignalAbort); RaiseException(EXC_SIGNAL_ABORT, 0, 0, 0); } ... // at program start: signal(SIGABRT, onSignalAbort);
"Pure Virtual" Method Calls

Ever see a program fail with that useless "pure virtual function call" error message? This happens when a base class's constructor tries to call a pure virtual method implemented by a derived class. Since base class constructors run before derived class constructors, the compiler fills the vtable for the derived class with references to _purecall, a function normally defined by the CRT. _purecall() aborts the process, sidestepping our crash reporting. Code might better elucidate this situation:
struct Base; void foo(Base* b); struct Base { Base() { foo(this); } virtual void pure() = 0; }; struct Derived : public Base { void pure() { } }; void foo(Base* b) { b->pure(); } Derived d; // boom
The fix is simple: just define a _purecall
that shadows the CRT implementation:
int __cdecl _purecall() { RaiseException(EXC_PURE_CALL, 0, 0, 0); return 0; }
Throwing an Exception from a Destructor During Unwind
C++ is aggressive about making sure you don't throw an exception while another exception is in the air. If you do, its default behavior is to terminate your process. From MSDN: If a matching handler is still not found, or if an exception occurs while unwinding, but before the handler gets control, the predefined run-time function terminate is called. If an exception occurs after throwing the exception, but before the unwind begins, terminate is called.
To convert calls to terminate()
(and unexpected()
, for completeness) into structured exceptions, override the terminate handler with set_terminate (and set_unexpected):
void onTerminate() { RaiseException(EXC_TERMINATE, 0, 0, 0); } void onUnexpected() { RaiseException(EXC_UNEXPECTED, 0, 0, 0); } // at program start: set_terminate(onTerminate); set_unexpected(onUnexpected);
Standard C++ Library Index/Iterator Error with Checked Iterators Enabled
The IMVU client is compiled with _SECURE_SCL
enabled. Increased reliability from knowing exactly where failures occur is more important than the very minor performance hit of validating all iterator accesses.
There are two ways to convert invalid iterator uses into reported exceptions. The easiest is compiling with _SECURE_SCL_THROWS=1
. Otherwise, just install your own invalid_parameter handler with _set_invalid_parameter_handler
.
Stack Buffer Overflow (with /GS enabled)
By default, Visual C++ generates code that detects and reports stack buffer overruns. This prevents a common class of application security holes. Unfortunately, the stock implementation of this feature does not allow you to install your own handler, which means you can't report any buffer overruns.
Again, we can shadow a CRT function to handle these failures. From C:\Program Files\Microsoft Visual Studio 8\VC\crt\src\gs_report.c
, copy the __report_gsfailure
function into your application. (You did install the CRT source, didn't you?) Instead of calling UnhandledExceptionFilter
at the bottom of __report_gsfailure
, call your own last-chance handler or write a minidump.
Testing Crash Reporting
Writing tests for the above reporting mechanisms is super fun. Take everything you're told not to do and implement it. I recommend adding these crashes to your UI somewhere so you can directly observe what happens when they occur in your application. Here is our crash menu:
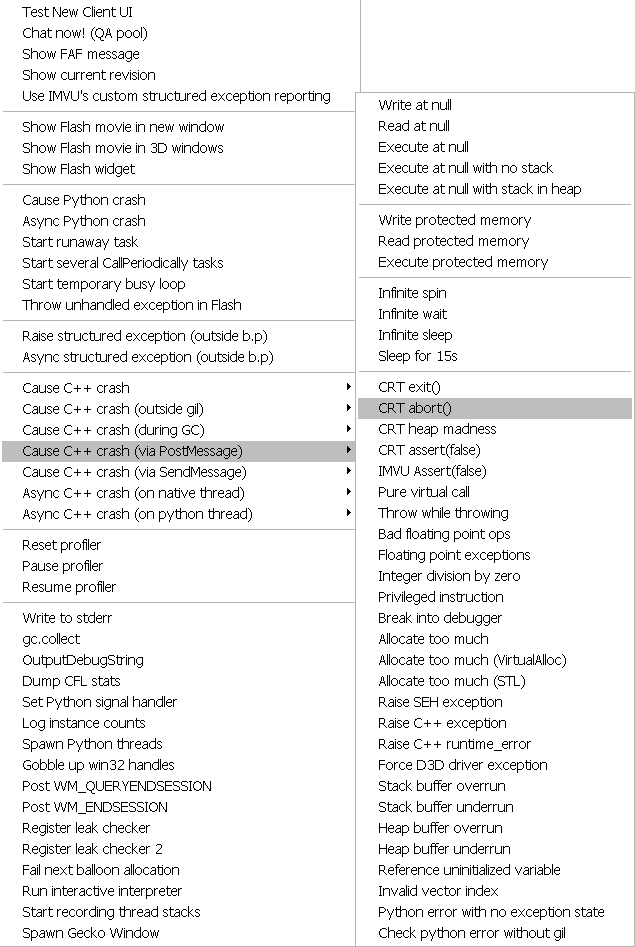
These types of failures are rare, but when we hit them, I'm glad we implemented this reporting.
See discussion on /r/programming.
i hve this problem when i tried to download the imvu version 418.0 and then i wanted to download the newer version of IMVU and it said MICROSOFT VISUAL c++ error failed and then i didnt know what to do i left it overnight and woke up in the morning to try downloading the new versoion of imvu again and the same MICROSOFT VISUAL c++ came out i need HELP ASAP to get my imvu back please
How we can shadow _reportgsfailure without recompiling CRT ? It's called inside CRT dll as far as I understand it. Please provide more details ...